In this tutorial, I am going to make an ajax based registration form in PHP with frontend and backend validation. I will be using PHP PDO for MYSQL database operations. For the front-end, I will be using bootstrap 5 with some custom CSS.
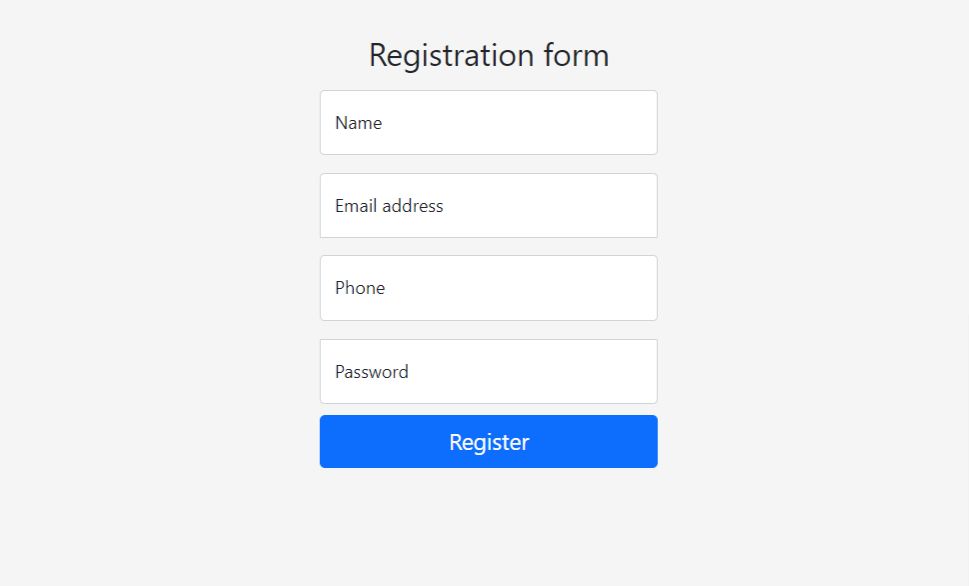
Jquery Ajax Registration Form with validation in PHP
- First of all, I will create a form using bootstrap 5 classes.
- Then I will apply frontend validation on the form submit
- If all fields will have values, the form data will post to the
form_ajax.php
file. Otherwise, the error field will be highlighted in red. Form_ajax.php
file will check backend validation. If any error appearsform_ajax.php
will return the error in JSON format.- If all goes well, the User record will save in the database. Using PHP PDO connection.
Create Database:
1 |
create database demo; |
Create MySQL Table:
Following is the create statement of site_users table.id
column is a primary key of the table. And email
column bind with unique key.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
CREATE TABLE `site_users` ( `id` INT(11) NOT NULL AUTO_INCREMENT, `name` VARCHAR(255) NULL DEFAULT NULL COLLATE 'latin1_swedish_ci', `email` VARCHAR(255) NULL DEFAULT NULL COLLATE 'latin1_swedish_ci', `password` VARCHAR(255) NULL DEFAULT NULL COLLATE 'latin1_swedish_ci', `phone` VARCHAR(255) NULL DEFAULT NULL COLLATE 'latin1_swedish_ci', `created_at` DATETIME NULL DEFAULT NULL, PRIMARY KEY (`id`) USING BTREE, UNIQUE INDEX `email` (`email`) USING BTREE ) COLLATE='latin1_swedish_ci' ENGINE=InnoDB ; |
CSS (custom.css):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
html, body { height: 100%; } body { display: block; align-items: center; padding-top: 0px; padding-bottom: 0px; background-color: #f5f5f5; } .form-signin { width: 100%; max-width: 330px; padding: 15px; margin: auto; } .form-signin .checkbox { font-weight: 400; } .form-signin .form-floating:focus-within { z-index: 2; } .form-signin input[type="email"] { margin-bottom: -1px; border-bottom-right-radius: 0; border-bottom-left-radius: 0; } .form-signin input[type="password"] { margin-bottom: 10px; border-top-left-radius: 0; border-top-right-radius: 0; } .bd-placeholder-img { font-size: 1.125rem; text-anchor: middle; -webkit-user-select: none; -moz-user-select: none; -ms-user-select: none; user-select: none; } @media (min-width: 768px) { .bd-placeholder-img-lg { font-size: 3.5rem; } } |
HTML (index.php):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
<html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Demo - Ajax Based registration form using PHP and MYSQL</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.1/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-F3w7mX95PdgyTmZZMECAngseQB83DfGTowi0iMjiWaeVhAn4FJkqJByhZMI3AhiU" crossorigin="anonymous"> <link rel="stylesheet" href="custom.css"> </head> <body class="text-center"> <main class="form-signin"> <form class="mt-5" id="registrationFrm" action="" method="POST"> <h1 class="h3 mb-3 fw-normal">Registration form</h1> <div id="response"></div> <div class="form-floating mb-3"> <input type="text" class="form-control" id="floatingName" placeholder="Name" name="name"> <label for="floatingName">Name</label> </div> <div class="form-floating mb-3"> <input type="email" class="form-control" id="floatingEmail" placeholder="name@example.com" name="email"> <label for="floatingEmail">Email address</label> </div> <div class="form-floating mb-3"> <input type="text" class="form-control" id="floatingPhone" placeholder="Phone" name="Phone"> <label for="floatingPhone">Phone</label> </div> <div class="form-floating"> <input type="password" class="form-control" id="floatingPassword" placeholder="Password" name="password"> <label for="floatingPassword">Password</label> </div> <button class="w-100 btn btn-lg btn-primary" type="submit" id="registerSubmit">Register</button> </form> </main> </body> </html> |
Jquery (index.php):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script> <script> $(document).ready(function(){ $("#registerSubmit").click(function(e){ e.preventDefault(); let emptyInputCount=0; $("#registrationFrm input").each(function(){ var input = $(this); if(input.val() == ''){ input.css('border-color','red'); emptyInputCount = 1; } else{ input.css('border-color','#ced4da'); } }); if(emptyInputCount == 0){ let getName = $("#floatingName").val(); let getPhone = $("#floatingPhone").val(); let getEmail = $("#floatingEmail").val(); let getPassword = $("#floatingPassword").val(); postObj = { name: getName, phone: getPhone, email: getEmail, password: getPassword, } $.ajax({ type: 'POST', url:'form_ajax.php', data:postObj, success: function(data){ //console.log(data); parseJson = JSON.parse(data); if(parseJson.success_msg) { $("#response").html('<div class="alert alert-success">'+parseJson.success_msg+'</div>'); } else { if(parseJson.error_count == 1) { $("#response").html('<div class="alert alert-danger">'+parseJson.error_msg+'</div>'); } else { let msg=''; for(i=0;i<parseJson.error_count;i++) { msg +='<div class="alert alert-danger">'+parseJson.error_msg[i]+'</div>'; } $("#response").html(msg); } } } }) } }); }); </script> |
PHP (form_ajax.php):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 |
<?php if(isset($_POST['name'],$_POST['phone'],$_POST['email'],$_POST['password'])) { $errorsArray = []; if(empty($_POST['name'])) { $errorsArray[] = 'Name is required'; } if(empty($_POST['phone'])) { $errorsArray[] = 'Phone is required'; } if(empty($_POST['email'])) { $errorsArray[] = 'Email is required'; } if(empty($_POST['password'])) { $errorsArray[] = 'Password is required'; } if (!filter_var(trim($_POST['email']), FILTER_VALIDATE_EMAIL)) { $errorsArray[] = "Invalid email format"; } if(count($errorsArray) > 0) { $errors['error_count'] = count($errorsArray); $errors['error_msg'] = $errorsArray; echo json_encode($errors); exit(); } $servername = "localhost"; $username = "root"; $password = ""; $database = 'demo'; try { $conn = new PDO("mysql:host=$servername;dbname=".$database, $username, $password); $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); } catch(PDOException $e) { echo json_encode(['error_msg' => $e->getMessage(), 'error_count' => 1]); exit(); } $name = htmlspecialchars(trim($_POST['name'])); $email = htmlspecialchars(trim($_POST['email'])); $phone = htmlspecialchars(trim($_POST['phone'])); $password = trim($_POST['password']); $hashPassword= password_hash($password,PASSWORD_BCRYPT,['cost'=>12]); $sql = "insert into site_users (name, email, password, phone, created_at) values(:name,:email,:password,:phone,:created_at)"; $prepare = $conn->prepare($sql); $params = [ 'name' => $name, 'email' => $email, 'password' => $hashPassword, 'phone' => $phone, 'created_at'=> date('Y-m-d H:i:s') ]; try{ $prepare->execute($params); echo json_encode(['success_msg' => 'User has been registered']); exit(); } catch(Exception $e){ echo json_encode(['error_msg' => $e->getMessage(),'error_count' => 1]); exit(); } } echo json_encode(['error_msg' => 'Access Denied','error_count' => 1]); exit(); ?> |
AAA High Quality Luxury Replica Rolex Watches Online Sale At rolex datejust replica.
Best hi quality replica rolex daytona watches is swiss watches, at https://www.daytonareplica.com sale 1:1 best fake rolex daytona watches, high-quality swiss movement.
Experience the allure of renowned Swiss watchmaking with our replica iwc, available at the best prices without compromising on quality.